Sudoku Solver
Solving Sudoku Puzzles in a GUI
I've always been a big fan of Sudoku. However, sometimes I come across one in the newspaper or a book that I cannot solve and do not have the solution to. I've always wanted a way of checking the answers. So I decided to built a program that would do just that.
The Algorithm
On the Github repo for this project. You'll see a Jupyter Notebook that shows the early stages of this project. And when I realised I started with too large of a scope. The end goal at the start of this project was to have a program that could read in Sudoku puzzles from an image, then product the solution.
This proved to be too much in one go. So instead, I created a .py file that would solve a Sudoku puzzle in the form of a 2D-array. This uses a traditional recursive approach.
- Find empty cell
- Try a number in the cell, and see if it's valid (check for duplicated in row, column and 3x3 block)
- If the number is valid, set that cell to the number and move to the next empty cell. If the number is not valid, try another number.
- Repeat this process until an empty cell has no valid inputs. I.e. all digits 1-9 are not valid in that cell.
- Set this cell to empty again
- Move back to previous cell being worked on and try the next number up
- Go to step 3
- Repeat until grid is filled
Below is the solver.py file that contains this process. This file is imported into the GUI file later on.
Next up was to experiment with Tkinter to create a GUI that displays the puzzle and solutions.
The GUI
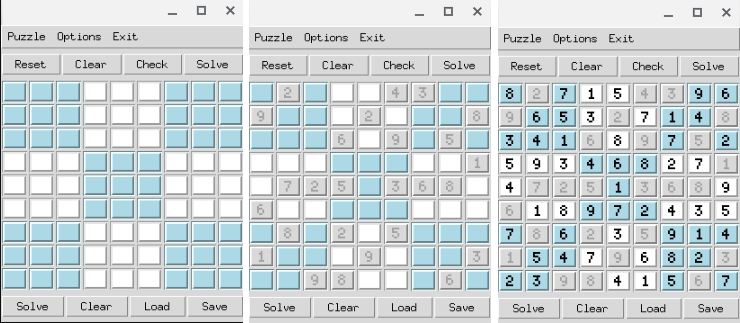
The idea was to create a simple grid of entry cells that allow the input of digits 1-9, and the display of the board in general.
My method was to create two Frame objects. One to house the board, and one to house the buttons. The Puzzle Frame containes a 9x9 grid of frames that each have a single Entry object in. These Entry objects validate the input and show the puzzle elements. They can also have their properties changed to show their state.
This GUI is a work in progess, and has some non-functional features. This is because it is going to become the GUI for the larger puzzle-solving project. Eventually this GUI will house the ability to play and solve Sudoku puzzles of any size. It will allow a user to input Sudoku puzzles from an image that is read by an OCR program I have written specifically for this.
There will also be other tabs for other puzzle types. The current next puzzle type is the Battleships puzzle.